Table of Contents
PL/I Programming Basics Part 2
Below is the continuation of PL/I programming basics from my previous article, if you would like check the previous one, Part-1 link is here. In this article, let’s discuss a little more advanced topics like various statements, arithmetic operations and other functions in PL/I.
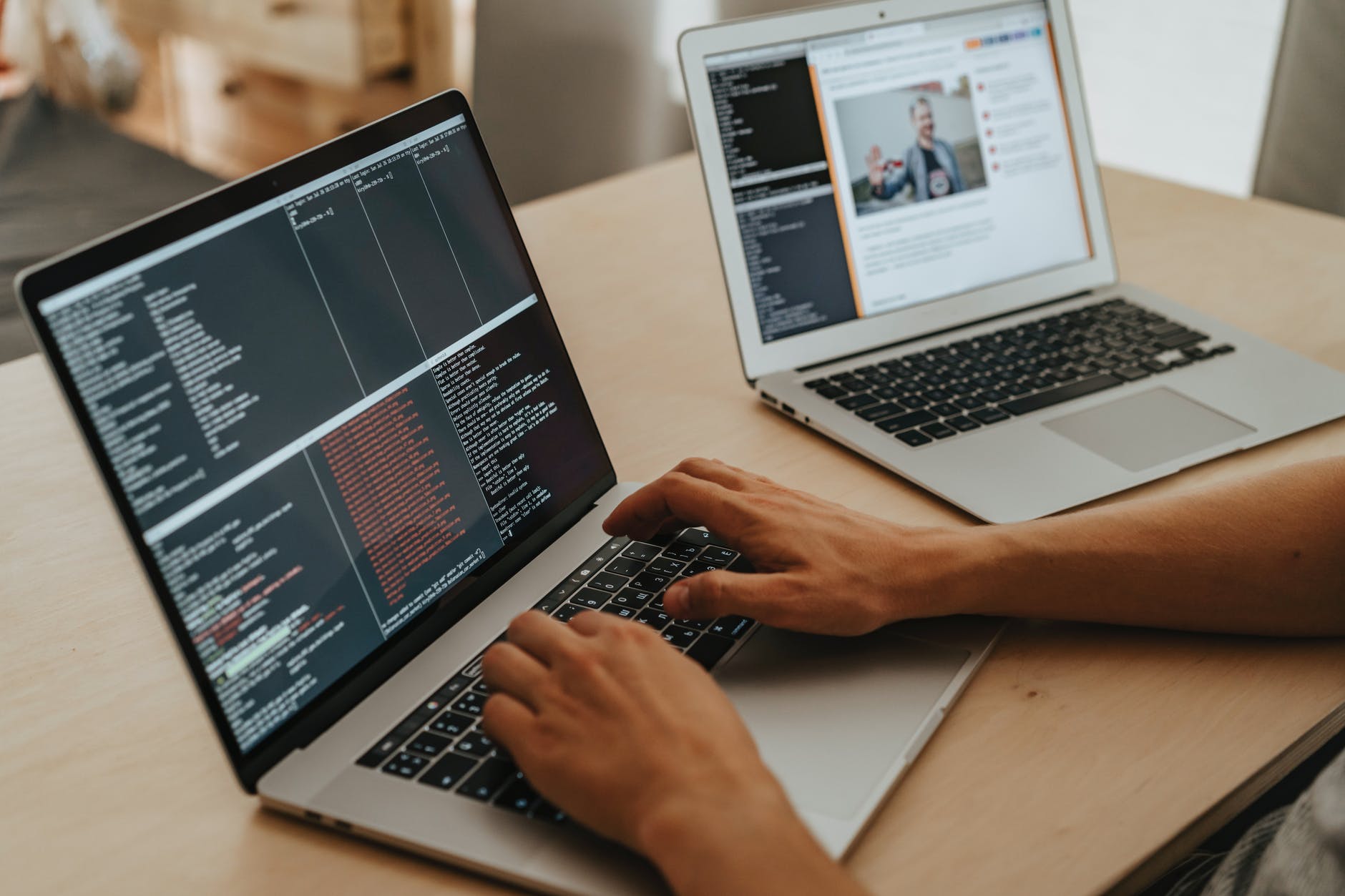
ASSIGNMENT STATEMENT
Used to assign some value to the identifier and used for computation purpose. i.e. The value of the expression on the right side of the equal symbol moved to the variable o the left side of the equal symbol.
Syntax :
{id1} = {id2} {arithmetic operator} {id3} / Expe. …….
{id1}, {id2}, {id3} = {id4} {arithmetic operator} …….
Examples:
a,b,c = x+y; a=(x*y)/z;
a = x**y;
a= (x+1)/y+1;
a=x**(j*2);
ARITHMETIC OPERATORS :
Allowable arithmetic operators are,
** – Exponential
* / / – Multiply / Division
+ / – – Addition / Subtraction
Order of Arithmetic Operations :
- 1. Exponential first – moves from right to left in an Exp.
- 2. Multiplication or Division – whichever appears first, moving from left to right in an Exp.
- 3. Addition or Subtraction – whichever appears first, moving from left to right in an Exp.
- 4. When parenthesis are specified, the inner most will be evaluated first and the next and soon.
- 5. Prefix operations are performed before infix operations.
- 6. If two or more operators of the highest priority appear in the same expr. the order of priority of those operators is from right to left.
- 7. Any expr. or element may be raised to power and power may have either +ve or -ve value, while exponent itself may be an expr. That will be evaluated first.
RELATIONAL OR COMPARISON OPERATORS :
GT – > – Greater Than
GE – >= – Greater Than or Equal to
LT – < – Less Than
LE – <= – Less Than or Equal to
EQ – = – Equal to
NE – != – Not Equal to
NG – !> – Not Greater Than
NL – !< – Not Less Than
LOGICAL OPERATORS :
NOT ( ), AND (&) & OR (|)
1 & 1 —-> 1 1 | 1 —-> 1 NOT 1 —-> 0
1 & 0 —-> 0 1 | 0 —-> 1 NOT 0 —-> 1
0 & 1 —-> 0 0 | 1 —-> 1
0 & 0 —-> 0 0 | 0 —-> 0
CONCATENATION:
The process of merging more than one Character string / Bit data is called Concatenation.
Examples :
FIRST_NAME = ‘ANAND’;
LAST_NAME = ‘BALASUBRAMANIAN KANDASWAMY’;
NAME1= FIRST_NAME || LAST_NAME;
NAME2= LAST_NAME || ‘,’ || FIRST_NAME;
FORMING PL/I STATEMENT :
LABEL: KEYWORD STATEMENT OPTIONS;
LABEL – Consists of the name of the procedure / modules. Most statements are not labeled.
KEYWORD – Consists of PL/I reserved words / key words and identifies the type of PL/I statement.
STATEMENT OPTIONS – are the programmer defined constants.
Each PL/I statement must end with the Semicolon, is the termination point of the statement.
PL/I is a free form, no special coding sheets are required. The following is the accepted IBM standard for typing programs.
Position 1 – Reserved for operating system.
Position 2 – 72 – May contain one or more PL/I statements or a part of statement.
Position 73-80 – May contain program identification name and/or a seq. no.
Note:
For program readability no more than one statement should appear on a line.
If the statement is lengthy, divide into smaller parts and code them on separate lines using indentation to highlight logical grouping of code.
When you have doubts use blanks i.e. GETLIST / GET LIST is having same meaning.
Blanks are not permitted in such character combination. i.e. > = is not equal to >=.
DECLARE STATEMENT in PL/I
The DECLARE Statement may appear anywhere following the PROCEDURE statement. It is a good practice to group the DECLARE Statement. together at the beginning of the program. Below are the needs if declare statements.
- When the programmer does not wish to use the data formats that are assigned by the compiler by default.
- When the information is supplied to the compiler that an identifier represents a array / structure.
- Ÿ When the input and output files are declared explicitly within the PL/I program.
Syntax :
DCL {pgmmer selected identifier} {data type} {position attribute}
Examples :
DCL PRICE DECIMAL FIXED (5,2);
| | |
| | Precision Attribute
Base Attribute Scale Attribute
BASE, SCALE & PRECISION ATTRIBUTES:
These attributes are used to describe the Characteristics of the data item. The scale Attribute can be declared first and then the Base attribute.
The precision attribute specifies the number of significant digits of the data and the decimal / binary alignment. From the above example Number of fractional part is 2 and Number on decimal or significant digits including the fractional digits.
Note :
If you are not specifying Base, Scale or Precision of the data items declared, the compiler will assume certain attributes by default. This may cause problems.
There can be any number of spaces between the attributes. But the precision attribute must follow the Scale or Base attribute.
The decimal part of the data item is including the fractional digits.
The precision never specified alone, becomes invalid precision declaration.
DCL QTY (10,0); /* ERROR */
Don’t specify fractions in the precision attribute for the floating point data.
DCL PI FLOAT DECIMAL (6,5); /* ERROR */
- Declared attributes Default Attributes
- DECIMAL FIXED (5,0)
- DECIMAL FLOAT (6)
- BINARY FIXED (15,0)
- BINARY FLOAT (21)
- DECIMAL FLOAT (6)
- BINARY FLOAT (21)
- FIXED DECIMAL (5,0)
- FLOAT DECIMAL (6)